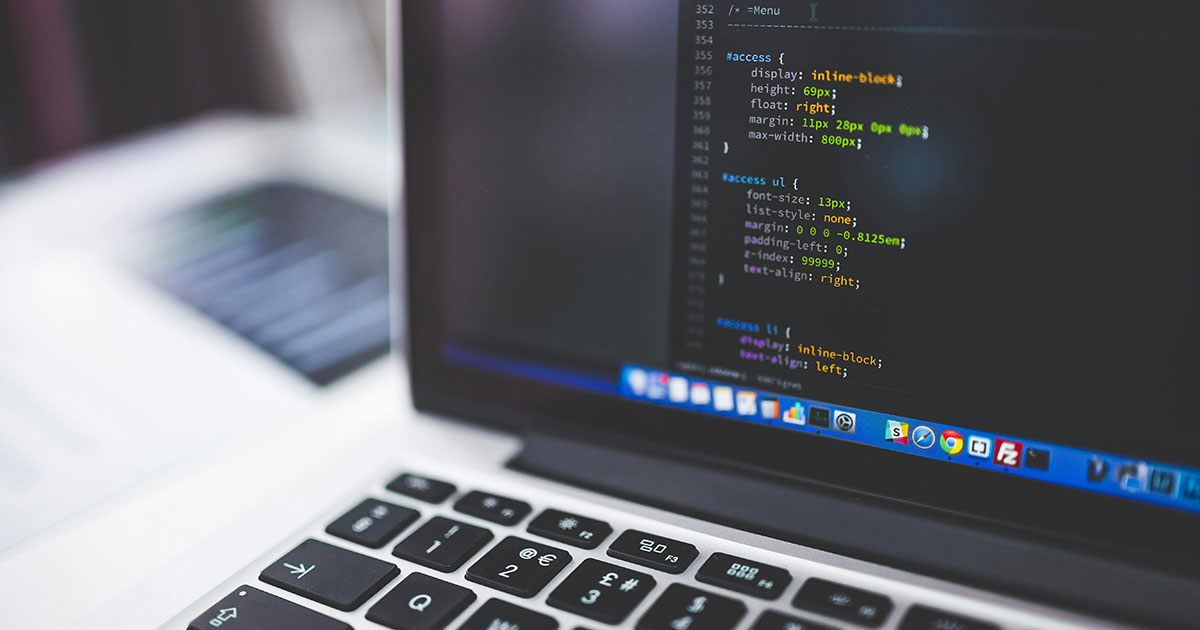
CSS Class Naming Conventions: Best Practices for Clean, Maintainable Code
One of the most important aspects of writing clean, maintainable CSS code is establishing clear and consistent CSS class naming conventions. Without a proper naming system, CSS files can quickly become chaotic, difficult to manage, and prone to conflicts. By following a well-structured naming convention, you can keep your stylesheets organized, reduce code duplication, and make collaboration easier for development teams.
In this guide, we’ll explore different CSS class naming conventions, including some of the most popular methods like BEM (Block, Element, Modifier), OOCSS (Object-Oriented CSS), and more. We’ll also provide best practices to help you choose the right approach for your project.
Why CSS Class Naming Conventions Matter
CSS class names are fundamental to how styles are applied across your web pages. A poorly structured naming system can lead to confusion, hard-to-read code, and unintended style conflicts.
Using clear and logical CSS class naming conventions offers several benefits:
- Readability: Descriptive class names make your CSS easier to understand and maintain, both for yourself and other developers who may work on the project later.
- Scalability: Well-structured naming conventions make it easier to scale and extend your CSS as your website or application grows.
- Avoid Conflicts: Proper naming helps prevent class name conflicts, especially in larger projects with many components and external libraries.
- Reusability: Consistent naming allows for the reuse of styles across multiple elements, reducing code duplication.
Common CSS Class Naming Conventions
There are several widely adopted CSS class naming conventions, each offering its own strengths depending on the scope and needs of your project. Below are some of the most popular methods:
1. BEM (Block, Element, Modifier)
BEM is one of the most commonly used CSS class naming conventions, particularly in large-scale projects. The goal of BEM is to make your CSS more modular, reusable, and maintainable by breaking down the UI components into logical sections: Blocks, Elements, and Modifiers.
How BEM Works:
- Block: The top-level component or parent. For example, a navigation menu might be a block (
.nav
). - Element: A component inside the block that has a specific function. It’s related to the block. For example, a navigation item inside
.nav
would be.nav__item
. - Modifier: A variation of the block or element. For instance, you might have a modifier to indicate the active state of a navigation item:
.nav__item--active
.
BEM Syntax:
/* Block */
.nav {
/* styles for the navigation block */
}
/* Element */.nav__item {
/* styles for the navigation items */
}
/* Modifier */.nav__item–active {
/* styles for the active navigation item */
}
Pros of BEM:
- Highly structured and easy to understand.
- Scalable and reusable across projects.
- Helps reduce specificity issues.
Cons of BEM:
- Class names can get quite long and verbose.
- May feel overkill for smaller projects.
2. OOCSS (Object-Oriented CSS)
OOCSS is a methodology that promotes the separation of structure and skin (or styling) to make your CSS more modular and reusable. With OOCSS, you aim to create reusable “objects” (like buttons, cards, or containers) that can be styled differently while keeping the underlying structure intact.
OOCSS Naming Example:
/* Structure (object) */
.card {
padding: 1rem;
border: 1px solid #ccc;
}
/* Skin (appearance) */.card-primary {
background-color: #007bff;
color: white;
}
Pros of OOCSS:
- Great for modular, reusable design components.
- Helps separate concerns (structure vs. appearance).
- Makes your CSS more DRY (Don’t Repeat Yourself).
Cons of OOCSS:
- May require careful planning to avoid conflicts.
- Some developers find it difficult to separate structure from styling.
3. SMACSS (Scalable and Modular Architecture for CSS)
SMACSS focuses on breaking down your CSS into different categories or rulesets to maintain scalability and avoid complexity. It categorizes CSS into Base, Layout, Module, State, and Theme rules.
SMACSS Categories:
- Base: Default styles for HTML elements (e.g., typography).
- Layout: Styles that define the page structure (e.g., header, footer).
- Module: Independent components or elements (e.g., buttons, forms).
- State: Styles that define the state of an element (e.g., active, hidden).
- Theme: Styles that define different themes (e.g., dark mode).
SMACSS Naming Example:
/* Base */
body, h1, p {
margin: 0;
padding: 0;
}
/* Layout */.layout-header {
background: #f5f5f5;
padding: 1rem;
}
/* Module */.btn {
padding: 0.5rem 1rem;
background-color: #007bff;
color: white;
}
/* State */.is-hidden {
display: none;
}
Pros of SMACSS:
- Highly scalable and modular.
- Helps create a consistent structure for larger projects.
Cons of SMACSS:
- Requires more initial setup and planning.
- The complexity of the methodology can be overkill for smaller projects.
4. Atomic CSS
Atomic CSS is a utility-first approach where each class represents a single property or style. Instead of creating components or modules, you apply individual classes to elements to define their styles.
Atomic CSS Naming Example:
<div class="p-4 bg-blue text-white">Hello, World!</div>
In this example:
p-4
defines padding.bg-blue
sets the background color.text-white
sets the text color.
Pros of Atomic CSS:
- Highly reusable and reduces specificity issues.
- Encourages consistency and reduces the need for complex custom components.
Cons of Atomic CSS:
- Can result in cluttered HTML with many utility classes.
- Difficult to maintain across larger projects with complex design requirements.
Best Practices for CSS Class Naming Conventions
Regardless of which CSS class naming convention you choose, here are some best practices to follow for clean and maintainable CSS:
1. Keep Class Names Descriptive
Use class names that clearly describe the purpose of the element or component. Avoid vague names like .box
or .item
—instead, use descriptive names like .nav__item
or .card-header
.
2. Use Hyphens or Underscores Consistently
Hyphens (-
) are the most common choice for separating words in CSS class names (e.g., .header-title
). However, some naming conventions, like BEM, use double underscores (__
) and double hyphens (--
). Whichever style you choose, be consistent.
3. Avoid Overly Specific Class Names
Class names should be specific enough to describe the element but not so specific that they become tied to a particular design or layout. For example, .button-blue-rounded
is too specific. Instead, use something like .btn-primary
.
4. Limit Nesting
Avoid excessive class nesting, which can lead to overly complex and hard-to-maintain code. Stick to a shallow hierarchy where possible to keep your CSS organized and easy to debug.
5. Use Semantic Class Names
Choose class names that reflect the content or purpose of the element, rather than its appearance. For example, instead of .red-btn
, use .btn-primary
or .btn-danger
, which conveys meaning rather than a specific style.
Choosing the Right CSS Class Naming Convention for Your Project
The best CSS class naming convention for your project depends on the size, complexity, and team working on the website. Here are some guidelines:
- For large-scale projects with many developers: Consider using BEM or SMACSS, as these conventions provide clear structures that can scale easily.
- For smaller or simpler projects: OOCSS or Atomic CSS might be more suitable, as they are easier to implement with fewer components.
- For utility-based designs: If your project relies heavily on reusable classes and utility-first design, Atomic CSS or frameworks like Tailwind CSS can work well.
Conclusion
Choosing the right CSS class naming convention is key to maintaining clean, scalable, and organized code. Whether you opt for BEM, SMACSS, OOCSS, or Atomic CSS, consistency is crucial for making your stylesheets easier to manage and extend. By adopting one of these naming conventions and following best practices, you’ll create a more maintainable codebase that’s easier to understand and collaborate on.
At Masthead Technology, we specialize in building efficient and maintainable websites. If you need help structuring your CSS or improving your website’s performance, reach out to us for professional web development and design services.
FAQs
1. What is BEM in CSS?
BEM stands for Block, Element, Modifier, and it’s a popular CSS class naming convention that helps create modular and reusable components.
2. Why are CSS class naming conventions important?
Class naming conventions help keep your CSS organized, readable, and maintainable, making it easier to work on projects over time and with multiple developers.
3. Which CSS naming convention should I use for a large project?
For large projects, BEM or SMACSS is highly recommended due to their scalability and structured approach.
4. Can I mix different CSS naming conventions in one project?
While it’s possible to mix naming conventions, it’s generally best to stick with one to ensure consistency and avoid confusion in your codebase.
5. What is the benefit of using Atomic CSS?
Atomic CSS promotes the use of small, reusable utility classes for styling elements, which reduces CSS specificity issues and makes it easier to maintain consistency across a project.